项目介绍
iBoxDB 是一个高能效的应用程序数据库,提供事务支持,使用数据库表的风格存取任意结构的文档对象数据,包含无须安装配置的 JAVA 与 .NET 数据库引擎。
iBoxDB 提供一个优雅设计的编程接口,让应用程序无缝地与数据引擎溶为一体, 你能方便地把它发布到移动设备, 桌面设备和服务器上, 然后让它帮助你存取各种有结构的,或者无结构的数据,具备保持数据一致性的能力。
双引擎数据库
支持 JAVA C# Android Unity3D Xamarin Mono ASP.NET Core Linux
特性
- 增删改查
- 键表与键值表
- 唯一或可重复的索引
- 唯一或可重复的复合索引
- SQL风格的数据查询语言
- 数据库事务支持
- 并发控制,多线程安全
- 多进程安全
- 内存管理
- 主从和多主的数据库热同步
- 支持磁盘永久保存和全内存快速保存
- 自动创建数据库文件
- 零配置, 复制直接运行, 全受控代码
- 动态列支持
- 带不同索引的多个数据类型能存到同一个表中
- 持久化 + 对象影射 + 缓存 + 嵌入式, 一站式解决方案
- Ason, 快速动态对象实例化
- 结果集支持 LINQ 与 STREAM
- 高性能无外部系统依赖
- 更新自增
- 查询追溯
- 两级事务隔离
- JAVA6+ .NET2+
示例
每个 BOX 都是一个完全隔离的独立数据空间
using(var box = db.Cube())
{
//select, insert, update, delete ...
var result = box.Commit();
}
try(Box box = db.cube()){
...
CommitResult r = box.commit();
}
普通对象操作
box["Member"].Insert(new Member() {
ID=box.NewId(),
Name = "Andy",
Password = EncodePassowrd("123")
}
);
Member m = new Member();
m.ID = box.newId();
m.setName("Kevin");
box.d("Member").insert(m);
动态列的文档数据
game["GameType"] = "ACT";
box["Product"].Insert(game);
game.put("GameType", "ACT");
box.d("Product").insert(game);
键-值风格的查询
box["Table", 2L].Select<Member>();
//支持复合键
box["Table2", 99, "ABC"].Select<Product>();
box.d("Table", 3L).select(Member.class);
//支持复合键
box.d("Table2", 88, "ABC").select(Product.class);
复合键支持
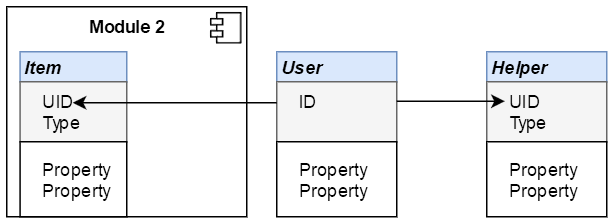
config.ensureTable(Item.class, "Item", "UID", "Type")
SQL 风格数据查询
//from TABLE where A>? & B<=? order by C limit 0,10
box.Select<Member>("from Member where Name==?", "MyName");
//from [table] where [condition]
// order by [field1] desc,[field2] limit [0,10]
//[Condition:] == != < <= > >= & | ( )
//[IFunction:] =[F1,F2,F3]
box.select(Member.class, "from Member where Name==?", "MyName");
弱类型支持
//使用强类型
box.select("from Table where Id > ?" , 1L);
//使用弱类型
box.select("from Table where Id > ?" , new Variant("1"));
自定义查询函数
box.Select<Member>("from Member where [Tags]", new Query("Value"));
兼容 LINQ (.NET)
from o in box.Select<Class>("from Class")
where o.Text.Contains(text)
select o;
兼容 Stream (Java8)
StreamSupport.stream(db.select("from Table").spliterator(), true)
.collect(Collectors.groupingBy((l) -> l.get("GroupID"),
Collectors.summingLong((l) -> (Long) l.get("Value"))));
Ason 和 原型对象
//定义原型, Id 是 Long
Ason prototype = new Ason("Id:", 0L, "Name:", "Guest");
Ason record = prototype.select();
//设置 Id 为 String,
record.set("Id", "123");
//自动转为定义原型的 Long
System.out.println("Output: " + record.get("Id").getClass());
//输出: class java.lang.Long
数据库热备份
auto.GetDatabase().CopyTo(new Mirror(bakAddr, bakRoot), buffer)
更新自增
作用域 | 触发条件 | 数据类型 | 数据来源 | |
---|---|---|---|---|
更新自增 | 索引 | 插入/更新 | 长整数 | Database NewId (MaxPos,1) |
主键自增 | 主键 | 插入 | 数字 | Table Max (ID)+1 |
查询追溯
线程 | 用法 | |
---|---|---|
查询追溯 | 不阻塞 | 读写不同的数据行 |
数据锁 | 阻塞 | 读写同一数据行 |
Snapshot-Serializable 两级事务
程序区域 | 隔离级别 |
---|---|
应用程序 | Snapshot |
数据库 | Serializable |
using(var box = auto.Cube()){
//Snapshot...
box.Commit( ()=>{
//Serializable...
});
}
数据类型支持
.NET | JAVA |
---|---|
|
|
ORM | |
|
|
数据持久层
IO |
---|
|
数据库路径设置
C# & JAVA, 把数据库文件放到项目工作目录外会有更好性能iBoxDB.LocalServer.DB.Root("/data/");
ASP.NET Cross Platform
iBoxDB.LocalServer.DB.Root(MapPath("~/App_Data/"));
Xamarin
iBoxDB.LocalServer.DB.Root(System.Environment.GetFolderPath(
System.Environment.SpecialFolder.Personal));
Unity3D
iBoxDB.LocalServer.DB.Root(Application.persistentDataPath);
Android
iBoxDB.LocalServer.DB.root(android.os.Environment.getDataDirectory()
.getAbsolutePath() + "/data/" + packageName + "/");
JSP WebApplication
@WebListener()
public class StartListener implements ServletContextListener {
@Override
public void contextInitialized(ServletContextEvent sce) {
String path = System.getProperty("user.home") + "/data/";
new File(path).mkdirs();
iBoxDB.LocalServer.DB.root(path);
}
}
查询方式
//查询
box.Select("from Member");
//查询,提前加载内存, 以 '*' 开始
box.Select("*from Member");
//查询追溯, 以 '!' 开始
box.Select("!from Member")
支持索引提升查询速度
config.EnsureIndex<Member>("Member", "Field1","Field2")
config.ensureIndex(Member.class, "Member", isUnique,"Field1","Field2")
box.Select("from Member where Field1 == ? & Field2 == ?")
快速入门 C# and JAVA
using IBoxDB.LocalServer;
var db = new DB();
db.GetConfig().EnsureTable<Record>("Table", "Id");
AutoBox auto = db.Open();
auto.Insert("Table", new Record { Id = 1L, Name = "Andy" });
var record = auto.Get<Record>("Table", 1L);
record.Name = "Kelly";
auto.Update("Table", record);
auto.Delete("Table", 1L);
import iboxdb.localserver.*;
DB db = new DB();
db.getConfig().ensureTable(Record.class, "Table", "Id");
AutoBox auto = db.open();
auto.insert("Table", new Record(1L, "Andy"));
Record record = auto.get(Record.class, "Table", 1L);
record.Name = "Kelly";
auto.update("Table", record);
auto.delete("Table", 1L);
安装使用
.NET: 在项目中引用 NETDB/iBoxDB.DLL
Java: 在项目中引用 JavaDB/iBoxDB.jar
与 MySQL 的性能参照
iBoxDB
Insert: 1,000,000 AVG: 47,016 objects/s
Update: 1,000,000 AVG: 25,558 objects/s
Delete: 1,000,000 AVG: 42,714 objects/s
MySQL
Insert: 1,000,000 AVG: 5,514 objects/s
Update: 1,000,000 AVG: 5,109 objects/s
Delete: 1,000,000 AVG: 6,044 objects/s
数据库同步,主从与多主
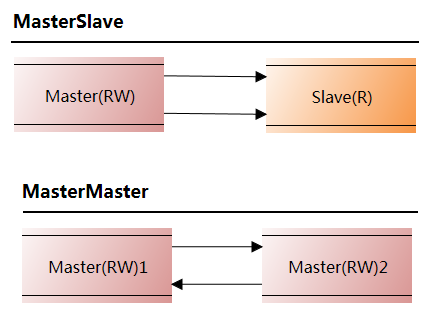
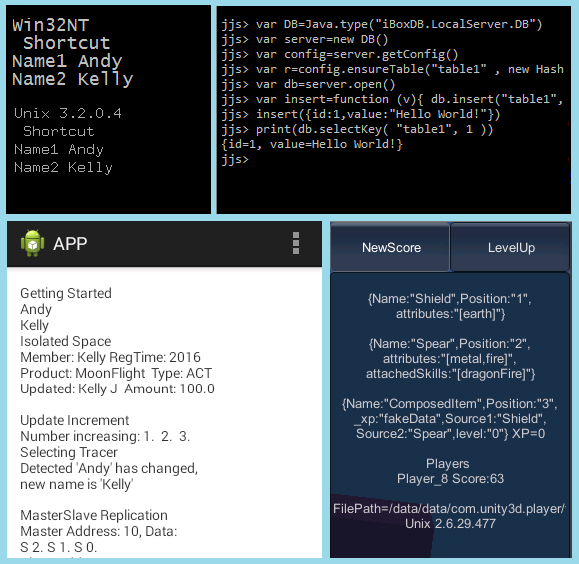